Easy theming using CSS variables
Here's a very easy theming for your website. The beauty of this technique is that you only need CSS.
I implemented it in a service for bookworms like myself.
This method is based on using the CSS variables (here's the spec). So make sure your browsers support it. Nowadays, the support is pretty solid, though.
I like it for a number of reasons:
- It's straightforward
- Pure CSS, thus, can be used with any framework
- Free previewing (see the image above)
- Changing themes is as easy as swapping classes on a root element
- Easy to debug/play around with using the DevTools
How does it work?
In CSS, we can define a variable (a correct term is "custom property") like this
body {
--page-bg: white;
--accent: dodgerblue;
--btn-bg: #DFCB52;
}
The defined variables are inherited by the children. We can, of course, use them in any part of the DOM tree within body
.
a {
color: var(--accent);
}
.btn {
background-color: var(--bg);
}
Now, since this is all good old CSS, we can override those variables by adding a class to the body.
.theme-violet {
--page-bg: white;
--accent: violet;
--btn-bg: #DFCB52;
// ... as many variables as you wish
}
.theme-dark {
--page-bg: #302E30;
--accent: #54A4F7;
--btn-bg: #112B3E;
// ...
}
// ... more themes
How do we change a theme? Just render the page with a corresponding theme class.
<body class="theme-violet">
<!-- ... -->
</body>
If you need to change a theme dynamically (to preview, for example), it's also very straightforward - change the class using your favorite framework, or vanilla JavaScript:
Then if I need to switch a theme, I can use a simple JS function.
function switchTheme(theme) {
document.body.classList = theme
}
switchTheme("theme-dark")
And that's basically it. A super-simple pure-CSS theming.
Where to go next
- It's never a bad idea to start with MDN.
- The W3C spec is pretty short and relatively easy to read.
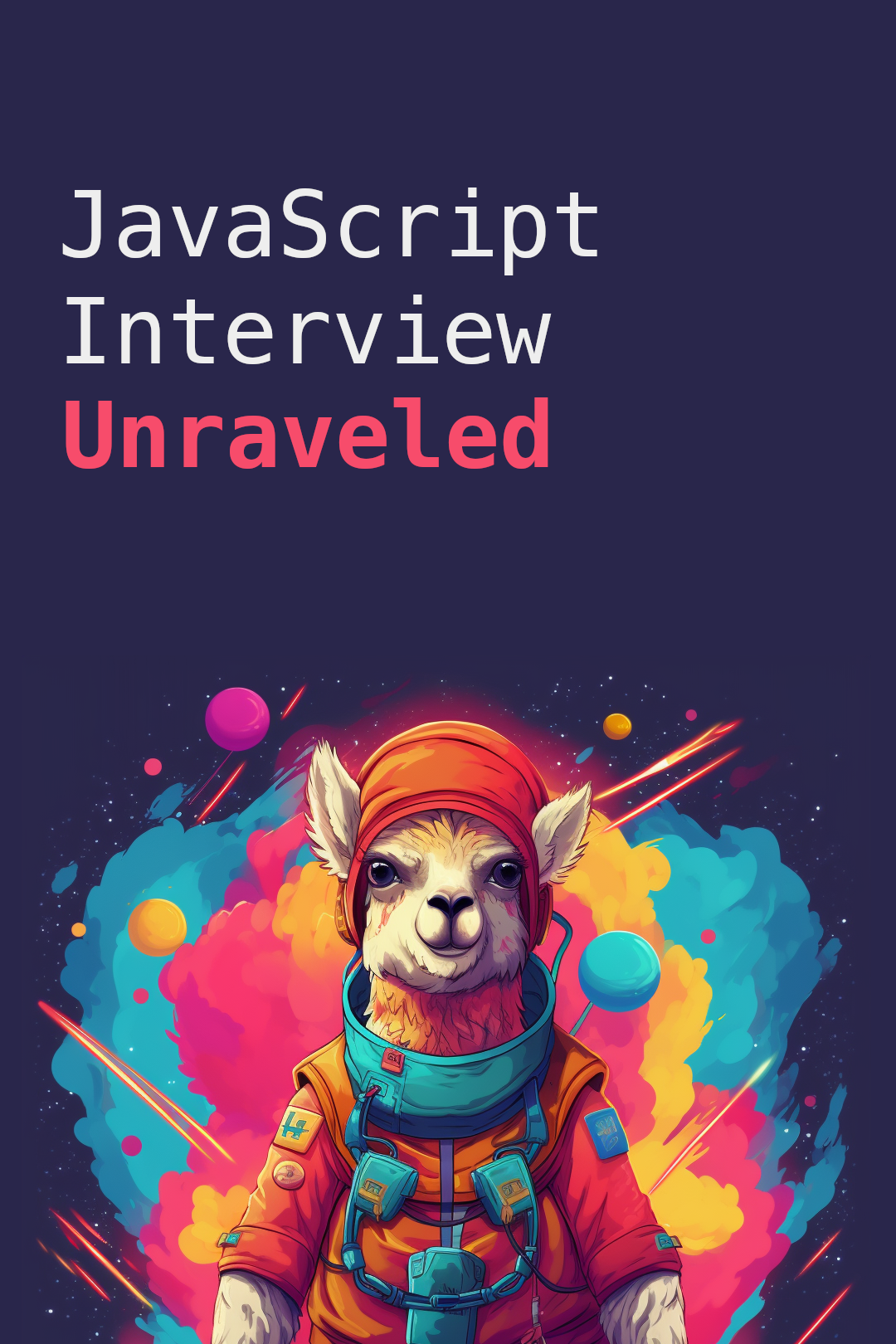