Upcoming ECMAScript features I'm excited about
ECMAScript spec train hasn't slowed down anyhow. There are still tons of great proposals waiting for their time.
Here are several of the upcoming ECMAScript features I'm particularly excited about.
Temporal
The date/time API has always been a weak point of JavaScript. Great libraries like moment.js (already deprecated) emerged to fix this, and they did great.
But now, finally, we got to a point where we can sum up our knowledge and experience and introduce a modern, robust date/time API into JavaScript standard library.
Temporal
is a new global object which acts as a module packed with utility functions (like Math
). The API is based on immutable objects, and there's excellent support for time zones and non-Gregorian calendars.
Here are some examples.
// parsing a string
const d = Temporal.Instant.from('1969-07-20T20:17Z')
// just plain date
Temporal.PlainDate.from({ year: 2000, month: 8, day: 2 });
// => 2000-08-02
// difference between two points
// in time in different time zones
const { from } = Temporal.ZonedDateTime
from('2020-03-08T11:55:00+08:00[Asia/Hong_Kong]')
.until(from('2020-03-08T09:50:00-07:00[America/Los_Angeles]'))
//=> PT12H55M
I recommend skimming through the cookbook to really realize the potential of this great API.
Array grouping
This small proposal adds two group
and groupToMap
to the Array's prototype.
Both methods are used to group items in an array by certain criteria (the closest relative is lodash's groupBy).
const users = [
{ name: "Jane" },
{ name: "John" },
{ name: "Tom" }
]
users.group(u => u.name.toLowerCase().charAt(0))
// {
// "j": [{name: "Jane"}, {name: "John"],
// "t": [{ name: "Tom" }]
// }
The groupToMap
works similarly but gives us a Map. It might come in handy when we need non-string keys.
Well, and there are other reasons to prefer Maps.
Array's change-by-copy (Stage 3)
What's wrong with Array's methods like reverse
, sort
, or splice
? Well, nothing, they are all great. The only problem is that they modify the array in place, making it harder to use them in an FP code.
This proposal introduces 4 new non-mutating methods on arrays (and also on typed arrays) which work similarly, but don't modify the source array: toReversed()
, toSorted()
, toSpliced()
, and with()
.
The last one is used to replace a single element in an array:
['j', 'a', 'n', 'e'].with(2, 'd')
//=> ['j', 'a', 'd', 'e']
Records and Tuples
Records and tuples are new primitive data structures similar to objects and arrays but deeply immutable.
const record = #{ name: "John", surname: "Smith" }
const tuple = #[1, 2, 3]
The syntax highlights the similarities between them and objects/arrays. All you have to do is to prefix it with #
.
Records and tuples can only contain other primitive values (including other records/tuples).
This limitation allows them to be deeply immutable.
It allows makes the equality comparison ===
work for them like for other primitives. If two records/tuples are structurally deeply equal, the ===
will return true
- and this is a big deal.
Introducing such data structures is a great way to improve the JavaScript functional capabilities (as immutability is crucial in the functional paradigm).
But this is quite a big topic, and I will return to it in a dedicated article.
Where to go next
Those were the great JavaScript proposals I can't wait for. Hope you like them too.
More proposals can be found on the official GitHub page.
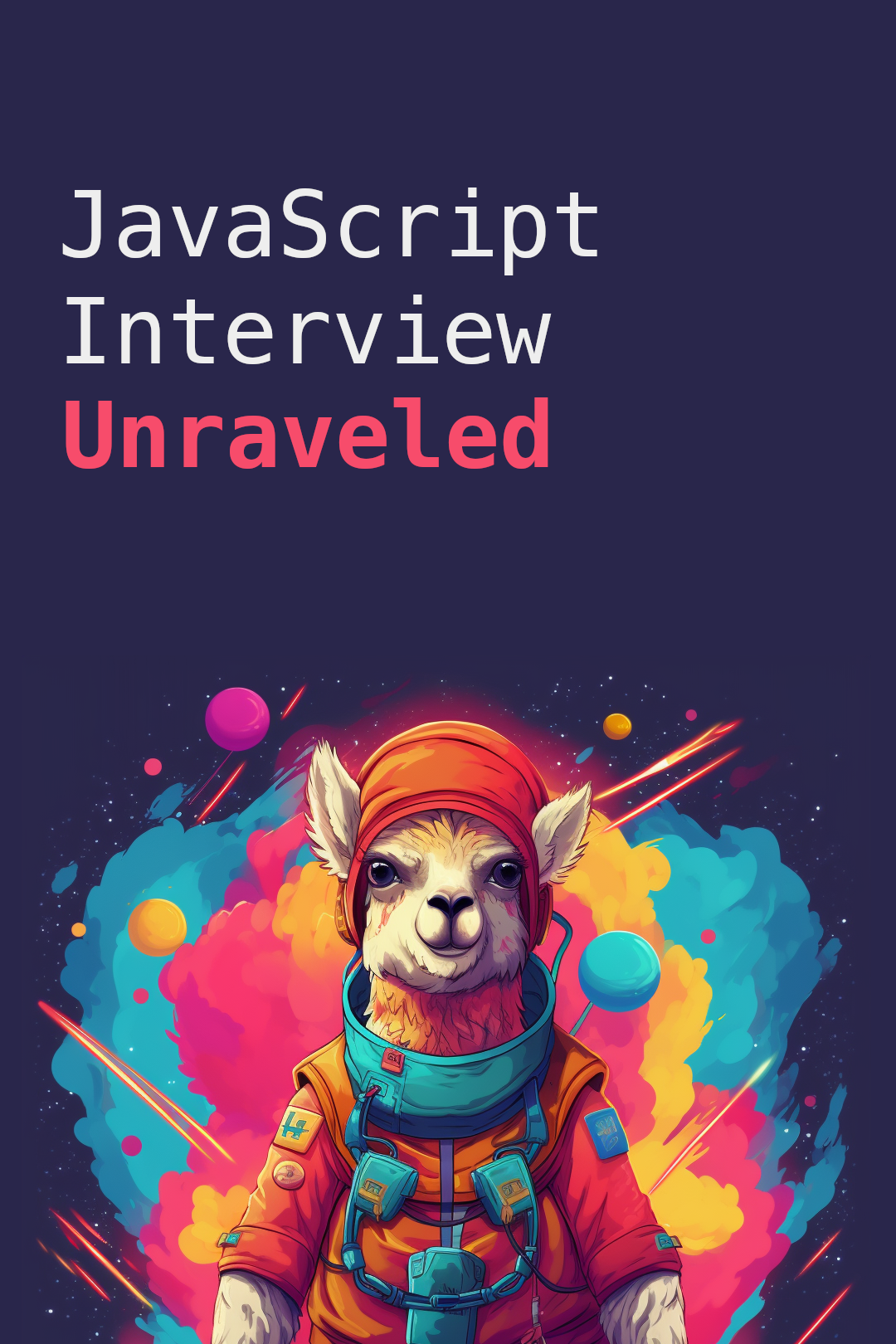