What are the differences between var, let, and const
In JavaScript, we can declare variables and optionally assign them values:
// declaring a variable with `var` keyword
var name;
// Declaring a variable and assigning a value
var name = "John";
Historically, var
was the only way to assign a variable, with let
/ const
introduced later as part of the ECMAScript 2015 (6th).
The var
declaration has some confusing properties when it comes to scope. On the other hand, const
and let
have cleaner scoping rules and usually should be preferred.
Let's see how they differ.
Variables declared with var
have a so-called "function-level scoping". They are accessible to any code within the same functions.
function testScope() {
var v = 123;
console.log(v); //=> 123
}
console.log(v); //=> Uncaught ReferenceError: v is not defined
When used outside of any function, it will assign values to the global scope. That means it will be accessible globally in any part of the code and available on the global object (e.g., window.myVar
).
Note that polluting global scope should be avoided (see the next question for details).
Functional scoping is opposed to block scoping when a variable is only visible within a block (that is, within curly braces {
/ }
). The let
and const
keywords declare variables with block scope.
Compare,
if (true) {
var name = "Emma";
let surname = "Stone";
}
console.log("name", name);
console.log("surname", surname); // ReferenceError: surname is not defined
Block-scoping is useful because it allows variables to only be visible within a block, thus preventing possible errors with overlapping variable names.
const
works similar to let
in terms of scoping. A difference between those two is that the format prevents re-assigning. You can only assign a value to that variable once, and it must be done at the time of declaration.
const age = 123;
age = 321; // TypeError: Assignment to constant variable.
let name = "John";
name = "Alex"; // No problem here
It's important to note that const
prevents re-assignment, but it doesn't make an object immutable.
const person = { name: "John" };
person.name = "Anna"; // perfectly valid
In practice, it's good to always default to const
. If you know that you'll need to re-assign the variable sooner, use let
. Avoid using var
(unless you specifically mean "function-level" scoping).
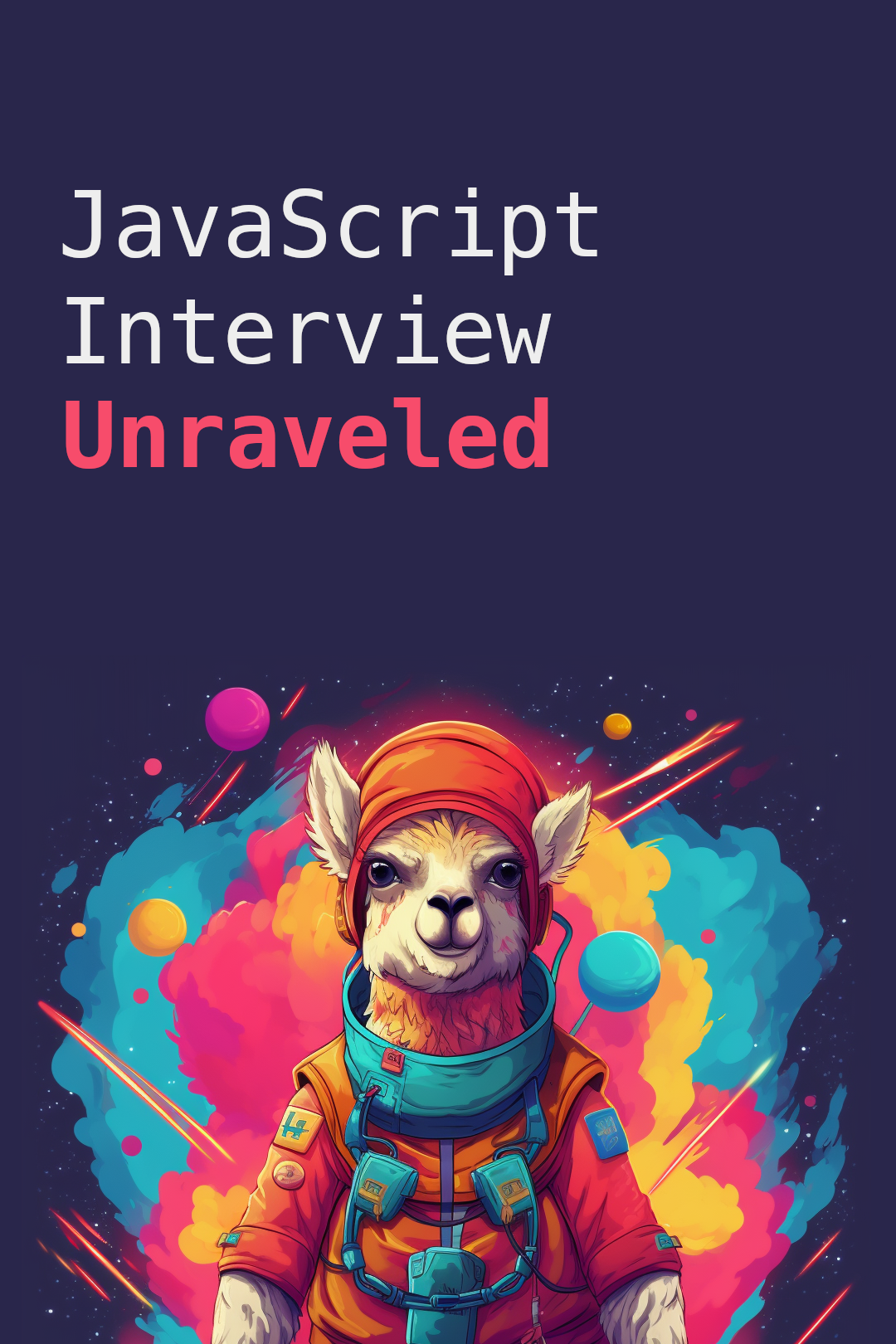